import { Avatar } from '@saas-ui/react'
export const AvatarBasic = () => {
return <Avatar name="Segun Adebayo" src="https://bit.ly/sage-adebayo" />
}
import { Avatar, AvatarGroup } from '@saas-ui/react/avatar'
<AvatarGroup>
<Avatar />
</AvatarGroup>
Use the size
prop to change the size of the avatar
import { HStack } from '@chakra-ui/react'
import { Avatar } from '@saas-ui/react'
export const AvatarWithSizes = () => {
return (
<HStack gap="3">
<Avatar size="xs" name="Sage" src="https://bit.ly/sage-adebayo" />
<Avatar size="sm" name="Sage" src="https://bit.ly/sage-adebayo" />
<Avatar size="md" name="Sage" src="https://bit.ly/sage-adebayo" />
<Avatar size="lg" name="Sage" src="https://bit.ly/sage-adebayo" />
<Avatar size="xl" name="Sage" src="https://bit.ly/sage-adebayo" />
<Avatar size="2xl" name="Sage" src="https://bit.ly/sage-adebayo" />
</HStack>
)
}
Use the shape
prop to change the shape of the avatar, from rounded
to
square
import { HStack } from '@chakra-ui/react'
import { Avatar } from '@saas-ui/react'
export const AvatarWithShape = () => {
return (
<HStack gap="4">
<Avatar
name="Dan Abramov"
src="https://bit.ly/dan-abramov"
shape="square"
size="lg"
/>
<Avatar
name="Sage Adebayo"
src="https://bit.ly/sage-adebayo"
shape="rounded"
size="lg"
/>
<Avatar
name="Random User"
src="https://images.unsplash.com/photo-1531746020798-e6953c6e8e04"
shape="full"
size="lg"
/>
</HStack>
)
}
The initials of the name can be used as a fallback when the src
prop is not
provided or when the image fails to load.
'use client'
import { HStack } from '@chakra-ui/react'
import { Avatar } from '@saas-ui/react'
export const AvatarWithFallback = () => {
return (
<HStack>
<Avatar name="Oshigaki Kisame" src="https://bit.ly/broken-link" />
<Avatar
name="Sasuke Uchiha"
src="https://bit.ly/broken-link"
colorPalette="teal"
/>
<Avatar src="https://bit.ly/broken-link" colorPalette="red" />
</HStack>
)
}
Use the outline*
props to add a ring around the avatar
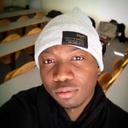


import { HStack, defineStyle } from '@chakra-ui/react'
import { Avatar } from '@saas-ui/react'
const ringCss = defineStyle({
outlineWidth: '2px',
outlineColor: 'colorPalette.500',
outlineOffset: '2px',
outlineStyle: 'solid',
})
export const AvatarWithRing = () => {
return (
<HStack gap="4">
<Avatar
name="Random"
colorPalette="pink"
src="https://randomuser.me/api/portraits/men/70.jpg"
css={ringCss}
/>
<Avatar
name="Random"
colorPalette="green"
src="https://randomuser.me/api/portraits/men/54.jpg"
css={ringCss}
/>
<Avatar
name="Random"
colorPalette="blue"
src="https://randomuser.me/api/portraits/men/42.jpg"
css={ringCss}
/>
</HStack>
)
}
Use the Group
component to group multiple avatars together
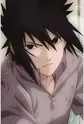
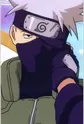
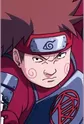
import { Avatar, AvatarGroup } from '@saas-ui/react'
export const AvatarWithGroup = () => {
return (
<AvatarGroup size="lg">
<Avatar
src="https://cdn.myanimelist.net/r/84x124/images/characters/9/131317.webp?s=d4b03c7291407bde303bc0758047f6bd"
name="Uchiha Sasuke"
/>
<Avatar
src="https://cdn.myanimelist.net/r/84x124/images/characters/7/284129.webp?s=a8998bf668767de58b33740886ca571c"
name="Baki Ani"
/>
<Avatar
src="https://cdn.myanimelist.net/r/84x124/images/characters/9/105421.webp?s=269ff1b2bb9abe3ac1bc443d3a76e863"
name="Uchiha Chan"
/>
<Avatar variant="solid" fallback="+3" />
</AvatarGroup>
)
}
When using the AvatarGroup
component, you can use the stacking
prop to
change the stacking order of the avatars
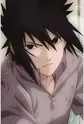
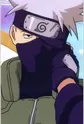
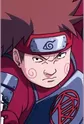
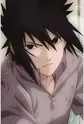
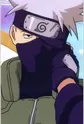
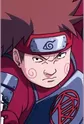
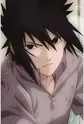
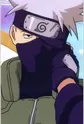
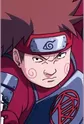
'use client'
import { Stack } from '@chakra-ui/react'
import { Avatar, AvatarGroup } from '@saas-ui/react'
export const AvatarGroupWithStacking = () => {
return (
<Stack>
<AvatarGroup size="lg" stacking="last-on-top">
{items.map((item) => (
<Avatar key={item.name} src={item.src} name={item.name} />
))}
<Avatar fallback="+3" />
</AvatarGroup>
<AvatarGroup size="lg" stacking="first-on-top">
{items.map((item) => (
<Avatar key={item.name} src={item.src} name={item.name} />
))}
<Avatar fallback="+3" />
</AvatarGroup>
<AvatarGroup size="lg" spaceX="1" borderless>
{items.map((item) => (
<Avatar key={item.name} src={item.src} name={item.name} />
))}
<Avatar fallback="+3" />
</AvatarGroup>
</Stack>
)
}
const items = [
{
src: 'https://cdn.myanimelist.net/r/84x124/images/characters/9/131317.webp?s=d4b03c7291407bde303bc0758047f6bd',
name: 'Uchiha Sasuke',
},
{
src: 'https://cdn.myanimelist.net/r/84x124/images/characters/7/284129.webp?s=a8998bf668767de58b33740886ca571c',
name: 'Baki Ani',
},
{
src: 'https://cdn.myanimelist.net/r/84x124/images/characters/9/105421.webp?s=269ff1b2bb9abe3ac1bc443d3a76e863',
name: 'Uchiha Chan',
},
]
Prop | Default | Type |
---|---|---|
colorPalette | 'gray' | 'gray' | 'red' | 'orange' | 'yellow' | 'green' | 'teal' | 'blue' | 'cyan' | 'purple' | 'pink' | 'accent' The color palette of the component |
size | 'md' | 'xs' | 'sm' | 'md' | 'lg' | 'xl' | '2xl' The size of the component |
variant | 'subtle' | 'solid' | 'subtle' | 'outline' The variant of the component |
shape | 'full' | 'square' | 'rounded' | 'full' The shape of the component |
asChild | boolean Use the provided child element as the default rendered element, combining their props and behavior. For more details, read our Composition guide. | |
ids | Partial<{ root: string; image: string; fallback: string }> The ids of the elements in the avatar. Useful for composition. | |
onStatusChange | (details: StatusChangeDetails) => void Functional called when the image loading status changes. | |
borderless | 'true' | 'false' The borderless of the component | |
as | React.ElementType The underlying element to render. | |
unstyled | boolean Whether to remove the component's style. |
Prop | Default | Type |
---|---|---|
asChild | boolean Use the provided child element as the default rendered element, combining their props and behavior. For more details, read our Composition guide. | |
name | string | |
icon | React.ReactElement |
Prop | Default | Type |
---|---|---|
asChild | boolean Use the provided child element as the default rendered element, combining their props and behavior. For more details, read our Composition guide. |